Reading CGMF Fission Events¶
[1]:
### initializations and import libraries
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.gridspec as gridspec
%matplotlib inline
%pylab inline
#import fission.py #-- my own library
sys.path.append('/Users/talou/git/evaluation-tools/')
import fission as fission
import fissionHistories as fh
Populating the interactive namespace from numpy and matplotlib
[2]:
### rcParams are the default parameters for matplotlib
import matplotlib as mpl
print ("Matplotbib Version: ", mpl.__version__)
mpl.rcParams['font.size'] = 18
mpl.rcParams['font.family'] = 'Helvetica', 'serif'
#mpl.rcParams['font.color'] = 'darkred'
mpl.rcParams['font.weight'] = 'normal'
mpl.rcParams['axes.labelsize'] = 18.
mpl.rcParams['xtick.labelsize'] = 18.
mpl.rcParams['ytick.labelsize'] = 18.
mpl.rcParams['lines.linewidth'] = 2.
font = {'family' : 'serif',
'color' : 'darkred',
'weight' : 'normal',
'size' : 18,
}
mpl.rcParams['xtick.major.pad']='10'
mpl.rcParams['ytick.major.pad']='10'
mpl.rcParams['image.cmap'] = 'inferno'
Matplotbib Version: 2.0.0
The default output of a CGMF run is an ASCII file that contains characteristics of fission events. Once the fissionHistories python class is uploaded, reading a CGMF output is straightforward:
[3]:
h = fh.FissionHistories ("cgmf-histories.dat", fmt="new")
Several python functions, part of the fissionHistories package, make it easy to analyze those events.
Accessing Fission Histories¶
Although we rarely need to access directly the fission histories, it is possible by typing:
[4]:
events=h.getFissionHistories()
[5]:
events[10]
[5]:
array([116, 45, 17.317, 17.0, -1, 108.176, 2, 7, list([0.093, 1.697]),
list([1.456, 4.31]),
list([0.652, 0.244, 1.28, 0.412, 0.784, 1.045, 0.069]),
list([0.652, 0.244, 1.28, 0.412, 0.784, 1.045, 0.069]), -4124.192,
2027.583, -1497.423, -4001.587, 2013.677, -1432.586,
list([array([-0.923, 0.259, -0.284]), array([-0.831, 0.006, -0.557])])], dtype=object)
This history first list the characteristics of the fission fragment in mass, charge, excitation energy, spin, parity, and kinetic energy. It then provides the number of neutrons and gamma rays emitted in this event, followed by their characteristics in energy and direction.
Accessing Fission Fragment Characteristics¶
Fission fragment characteristics can be obtained using accessors such as: getA(), getZ(), getNu(), etc.
[6]:
A=h.getA()
Z=h.getZ()
fig=figure(figsize(14,6))
plt.subplot(1,2,1)
plt.hist(A,bins=np.arange(min(A),max(A)),normed=True)
plt.xlabel("Fission Fragment Mass")
plt.ylabel("Probability")
plt.subplot(1,2,2)
plt.hist(Z,bins=np.arange(min(Z),max(Z)),normed=True)
plt.xlabel("Fission Fragment Charge")
plt.ylabel("Probability")
plt.tight_layout()
plt.show()
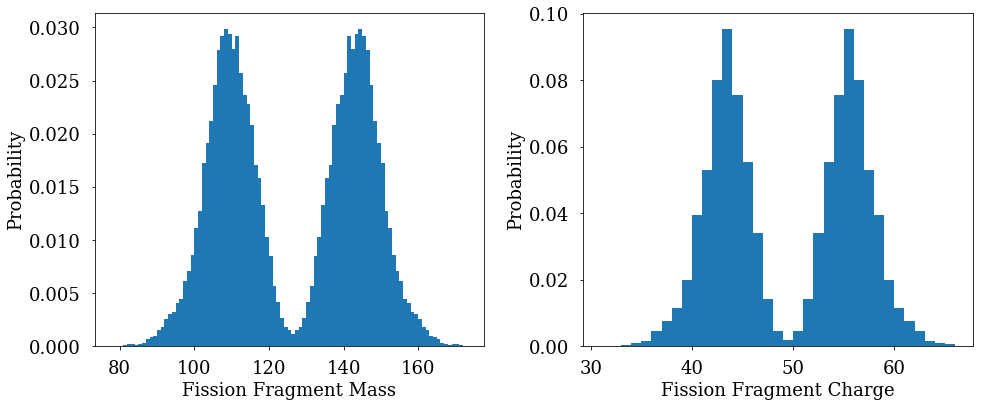
Plotting the initial excitation energy of the fragments as a function of its kinetic energy can be done by invoking getU() and getKE().
[14]:
U=h.getU()
KE=h.getKE()
bx=np.arange(min(U),max(U))
by=np.arange(min(KE),max(KE))
fig=figure(figsize(10,8))
plt.hist2d(h.getU(),h.getKE(),bins=(bx,by))
plt.xlim(0,40)
plt.xlabel("Initial Excitation Energy (MeV)")
plt.ylabel("Initial Fragment Kinetic Energy (MeV)")
plt.show()
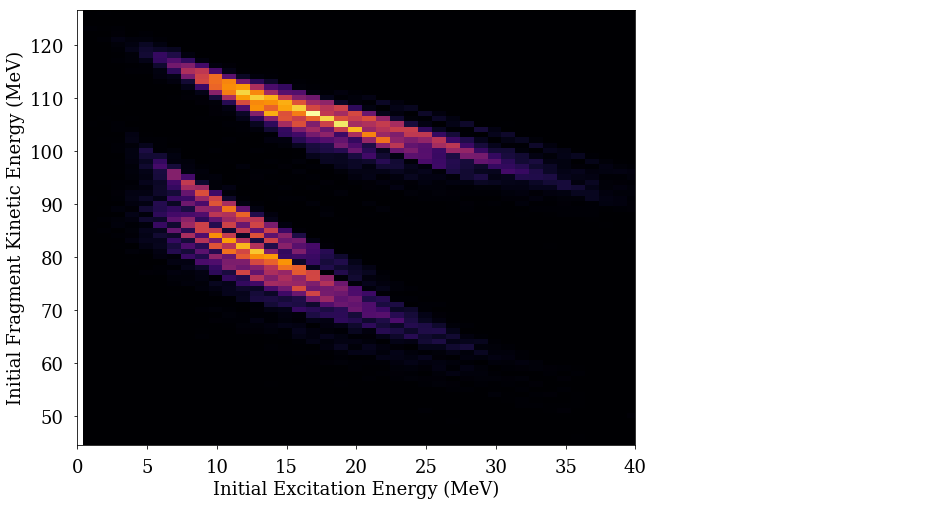
Summary table¶
A table summarizing the main characteristics of all fission events, fission fragments, neutrons and gamma rays can be generated by using the summaryTable() function.
[15]:
h.summaryTable()
[15]:
All Fragments | Light Fragments | Heavy Fragments | Pre-Fission | Total | |
A | 126.00 | 108.65 | 143.35 | ||
Z | 49.00 | 42.65 | 55.35 | ||
TXE / U (MeV) | 32.32 | 18.72 | 13.60 | ||
TKE / KE (MeV) | 185.83 | 105.58 | 80.26 | ||
J ($\hbar$) | 10.74 | 10.20 | 11.27 | ||
parity | 0.00 | -0.00 | 0.00 | ||
$\langle \nu\rangle$ | 3.75 | 2.12 | 1.64 | 0.00 | 3.75 |
$\langle \epsilon_n^{cm}\rangle$ (MeV) | 1.33 | 1.41 | 1.24 | 1.33 | 1.33 |
$\langle E_n^{lab}\rangle$ (MeV) | 2.10 | 2.36 | 1.77 | ||
$\langle \nu_\gamma\rangle$ | 9.31 | 4.70 | 4.61 | ||
$\langle \epsilon_\gamma^{cm}\rangle$ (MeV) | 0.74 | 0.75 | 0.74 | ||
$\langle E_\gamma^{lab}\rangle$ (MeV) | 0.74 | 0.75 | 0.74 |